It is the process of deriving two or more classes from single base class. And in turn each of the derived classes can further be inherited in the same way. Thus it forms hierarchy of classes or a tree of classes which is rooted at single class.
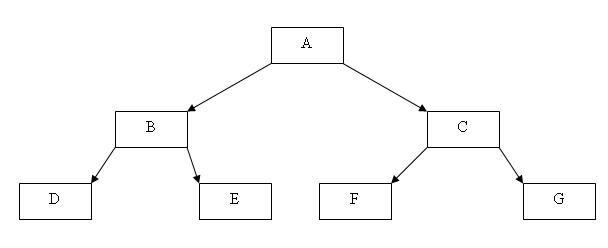
Here A is the base class from which we have inherited two classes B and C. From class B we have inherited D and E and from C we have inherited F and G. Thus the whole arrangement forms a hierarchy or tree that is rooted at class A.
The syntax and example for hierarchical inheritance in case of C++ is given below:
Syntax:
class A
{
-------
-------
};
class B : visibility_label A
{
-------
-------
};
class C : visibility_label A
{
-------
-------
};
class D : visibility_label B
{
-------
-------
};
class E : visibility_label B
{
-------
-------
};
class F : visibility_label C
{
-------
-------
};
class G : visibility_label C
{
-------
-------
};
Here the visibility_label can be private, protected or public. If we do not specify any visibility_label then by default is private.
class person
{
private:
char name[30];
int age;
char address[50];
public:
void get_pdata ( )
{
cout<<"Enter the name:";
cin>>name;
cout<<"\nEnter the address:";
cin>>address;
}
void put_pdata ( )
{
cout<<"Name: "<<name;
cout<<"\nAddress: "<<address;
}
};
class student : private person
{
private:
int rollno;
float marks;
public:
void get_stdata ( )
{
get_pdata ( );
cout<<"\nEnter roll number:";
cin>>rollno;
cout<<"\nEnter marks:";
cin>>marks;
}
void put_stdata ( )
{
put_pdata ( );
cout<<"\nRoll Number: "<<rollno;
cout<<"\nMarks: "<<marks;
}
};
class faculty : private person
{
private:
char dept[20];
float salary;
public:
void get_fdata ( )
{
get_pdata ( );
cout<<"\nEnter department:";
cin>>dept;
cout<<"\nEnter salary:";
cin>>salary;
}
void put_fdata ( )
{
put_pdata ( );
cout<<"\nDepartment: "<<dept;
cout<<"\nSalary: "<<salary;
}
};
void main ( )
{
student stobj;
faculty facobj;
clrscr ( );
cout<<"Enter the details of the student:\n";
stobj.get_stdata ( );
cout<<"\nEnter the details of the faculty member:\n";
facobj.get_fdata ( );
cout<<"\nStudent info is:\n";
stobj.put_stdata ( );
cout<<"\nFaculty Member info is:\n";
facobj.put_fdata ( );
getch ( );
}
Here ‘person’ is the base class from which ‘student’ and ‘faculty’ classes have been derived privately so the public members of the ‘person’ class will become private members of the ‘student’ class and ‘faculty’ class. But the private members will not be inherited.